Access Policies
Introduction
Access Policies control the circumstances in which data can be retrieved or edited. Practically, access policies are functions that receive contextual data and return true or false according to whether access is allowed or denied. Default context is generated from the server. The server context can be combined with additional context, which can be sent from the client.
Access Policies are used in three places in UserClouds:
- Every accessor (read API) is associated with an access policy that controls access for each target user record, and filters the records in the response accordingly
- Every mutator (write API) is associated with an access policy that governs whether the write is allowed
- Every token is associated with an access policy that governs the circumstances in which the token can be exchanged for the original data ("resolved")
Access policies allow you to govern data access centrally, even giving you the ability to modify contracts on data use after tokenized data is shared.
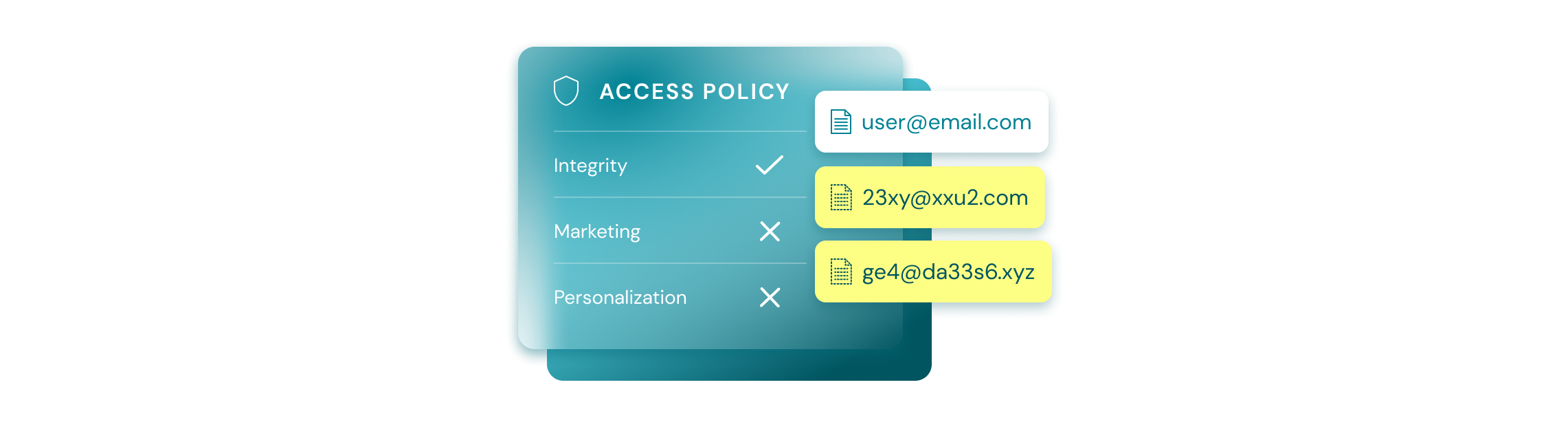
Access policies give you central, fine-grained control and visibility over sensitive data access. Policies can evaluate purpose, identity, authorization, location, expiration timelines and much more.
Access policies can be as simple as "always allow resolution" to complex Javascript evaluations including locations, credentials and purpose.
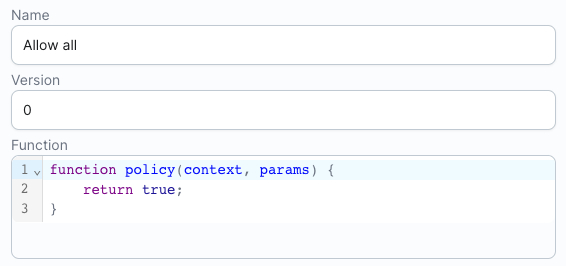
The simplest access policy returns true (i.e. Access Allowed) in all circumstances.
Examples
Let’s look at four possible access policies applied to a phone number token, to see how they work.
Example Policy | Use case |
---|---|
Only allow resolution for integrity and operational purposes | Marketing department cannot resolve phone number for SMS-based marketing |
Only allow resolution by users with role = employee | Contractor/third-party engineers cannot download phone number |
Only allow resolution after 30 days for fraud purposes | Phone number is effectively deleted from all systems after 30 days, but retained for fraud investigation |
Only allow resolution on trusted IP addresses | Phone number cannot be downloaded outside of company VPN |
External calls
Access policies can be configured to make calls to external systems. This includes other UserClouds systems (like Authentication and Authorization), your owned-and-operated systems and third party providers. This enables you to make access judgments like:
- Is the calling employee calling from a trusted IP address?
- Does the calling user have
view
permissions on the target user? - Does the calling user have an
admin
role? - Does this support rep have an open ticket for the target user in Zendesk?
What they are
An access policy consists of:
Name
Description
id
- a unique identifier, which can be used as a reference when creating accessors and tokensfunction
- a function with the signaturefunc(token Token, parameters Object, context Object)
, where context is passed in from the ResolveToken() call.parameters
- a static JSON object (not containing PII) that is available at runtime, allowing you to parameterize and reuse functions like "allow access only from IP range X-Y"
Managing access policies
UserClouds has several built-in access policies for common use cases, like role-based and time-based expiration of data. However you can also create custom policies, in two ways:
- Call the CreateAccessPolicy() API
- Compose a new policy from existing policies and parametrizable templates in the UserClouds Console
To learn more about creating access policies, see our How to Guide on Creating Access Policies.
Updated 8 months ago