Configure an accessor
Introduction
Accessors are configurable APIs that allow a client to retrieve data from the user store. Accessors are intended to be use-case specific. For example, you might configure two separate accessors GetEmailForMarketing and GetEmailForAuthentication. Accessors enforce data usage policies and minimize outbound data from the store for their given use case
Creating an Accessor
You can set up an accessor via the User Store page in the UserClouds Console, or using the CreateAccessor API. There are five steps to define an accessor:
- Name and describe the accessor
- Set the purpose of the accessor
- Choose which records and columns it will return data for
- Define how it will transform outbound data
- Define the circumstances in which the accessor can be used
Create an Accessor in UserClouds Console
To create an accessor, go the User Store page (accessible from the sidebar in UserClouds Console) and click Create Accessor.
1. Name and describe the accessor
Accessor names are used in your codebase (to call the API) and in your audit log (to understand whether data usage aligns with user consents). Use the names to describe what the API does and why it exists. A good go-to structure describes the transformed data and the purpose, e.g. GetAgeGroupForDataScience, GetPhoneNumberForMarketing or GetLast4OfPhoneForCustomerService.
2. Set the purpose of the accessor
Next, select the data processing purpose for which the accessor will be called. The accessor will filter out all user records and data values where consent for this purpose has not been gathered. You can learn more about how filtering for consent works here.
You can select a purpose from your existing purposes in the dropdown. To add a new purpose, return to the User Store page (accessible from the sidebar) and click "Add Purpose" at the bottom of the page.
3. Choose which records and columns the accessor will return data for
Next, pick the user records and columns the accessor will return data for. Specify the user records by writing a selector, a SQL-like clause, like {ID} = ANY(?) OR {Email} LIKE ?. In selectors, each ? represents a parameter that is passed in an array, at execution time. This parameter can be a single value, or an array of possible values. For more info on selectors, see Selectors.
Add columns by selecting from the dropdown and clicking "Add Column".
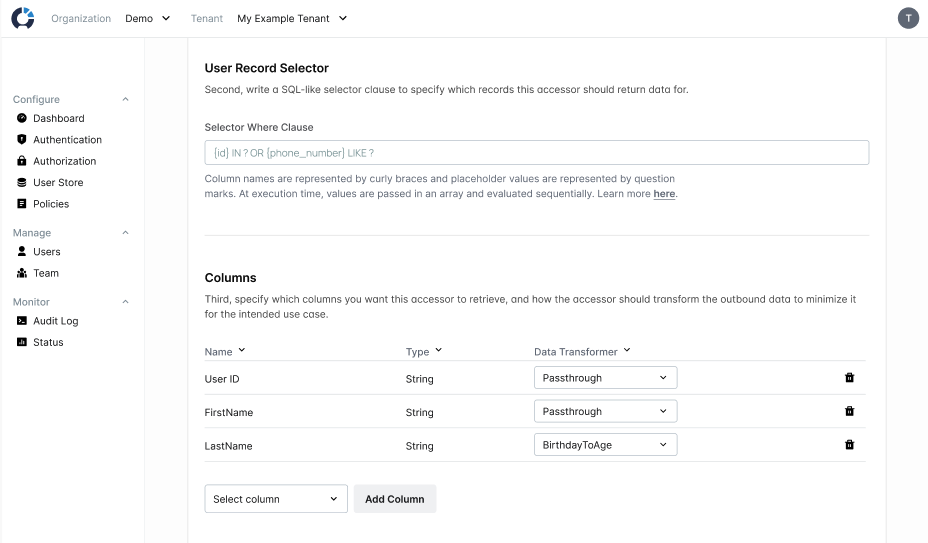
The User Record Selector and Columns are used to define which data the accessor will retrieve from the store.
4. Define how the accessor will transform outbound data
Next, you must define how the accessor will transform each column's data on the way out of the store. Choose one data transformer per column, using the dropdowns in the columns table that you create (see picture above). You can select a PassThrough transformer, which will pass the data through unmodified. If you select a tokenizing Transformer, you will also need to specify a token resolution policy, determining when the token can be exchanged to the original data.
Types of transformer
There are four types of transformers:
- PassThrough Transformers pass through the raw data, unchanged
- Data Transformers mask, categorize, inject noise or group raw data. They are used to retain the minimum characteristics of a piece of raw data for a particular task, without being resolvable back into the raw data.
- Tokenize By Value Transformers create a resolvable token with an associated access policy (called the token resolution policy). If the value of the raw data later changes, the token will resolve to the value of the data at the point of transformation.
- Tokenize By Reference Transformers create a resolvable token with an associated access policy (called the token resolution policy). If the value of the raw data later changes, the token will resolve to the latest value of the data. Tokens generated by reference also respect user consent changes.
Matching data types
Only transformers with an input type that can represent the data type of the column may be used. As such, the input type of the transformer must either exactly match the data type of the column, or be a more generalized form of that data type. For example:
- A transformer with the same input type as the column data type can be used
- A transformer with an input type of
string
can be used for a column with any valid data type, since we can always represent a data type as a string. - A transformer with input type
timestamp
can be used on a column with data typetimestamp
ordate
- A transformer with input type
date
cannot be used on column with data typeboolean
The type that is finally returned by the transformer is the output type of the transformer. The transformer will validate the output to ensure it can be represented as the specified output type.
Transforming array columns
Transformers should always be written to act on a single piece of data. UserClouds natively handles the case where the associated column is an array column, calling the specified transformer on each array element and recombining the results into an array.
For more information on transformers, see Transformers. For more information on creating transformers, see our How To Guide on Creating Transformers.
5. Define the circumstances in which the accessor can be used
Finally, select an access policy for the accessor. The access policy controls whether the accessor can retrieve data for each target user record, and filters the records in the response accordingly. It is how you enforce privacy and data security rules at the boundary of the store. The simplest access policy returns true
(access allowed) for all user records. It is possible to create much more fine-grained policies. In this interface, you can:
- Choose a single, pre-existing policy
- Choose and parametrize a pre-existing access policy template
- Write and parametrize a new policy template
- Compose a new policy by taking any of the above actions multiple times and combining those policies with
AND
orOR
logic
For more information on what access policies do, see Access Policies. For more information on creating access policies, see our How To Guide on Creating Access Policies.
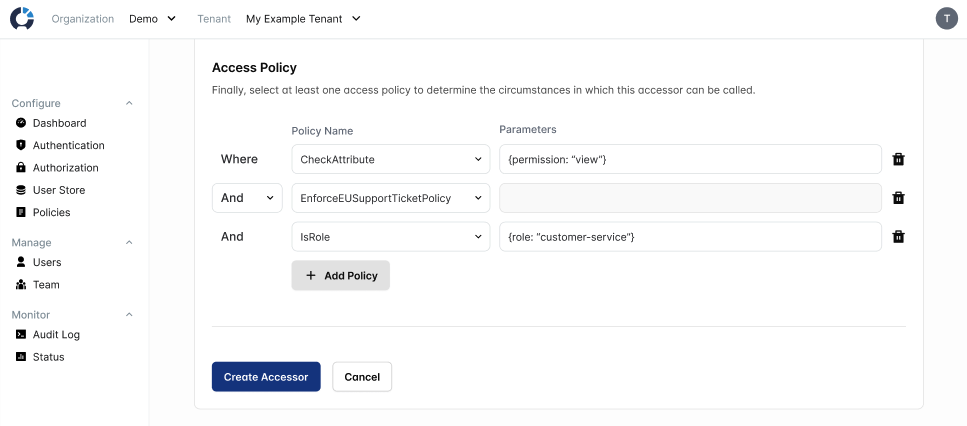
The access policy controls whether the can retrieve data for each target user record, and filters the records in the response accordingly
Nice job! You can now click Save to create your accessor. You can learn how to invoke this accessor in the next article.
Create an Accessor via the API
To learn how to create an accessor via the UserClouds API, see our [API Reference](https://docs.userclouds.com/reference/post_userstore-config-accessors).
Updated 3 months ago